Boost Application Speed with Redis Caching
Learn how Redis caching can dramatically speed up your applications, reduce server load, and create smoother user experiences even during high traffic periods.
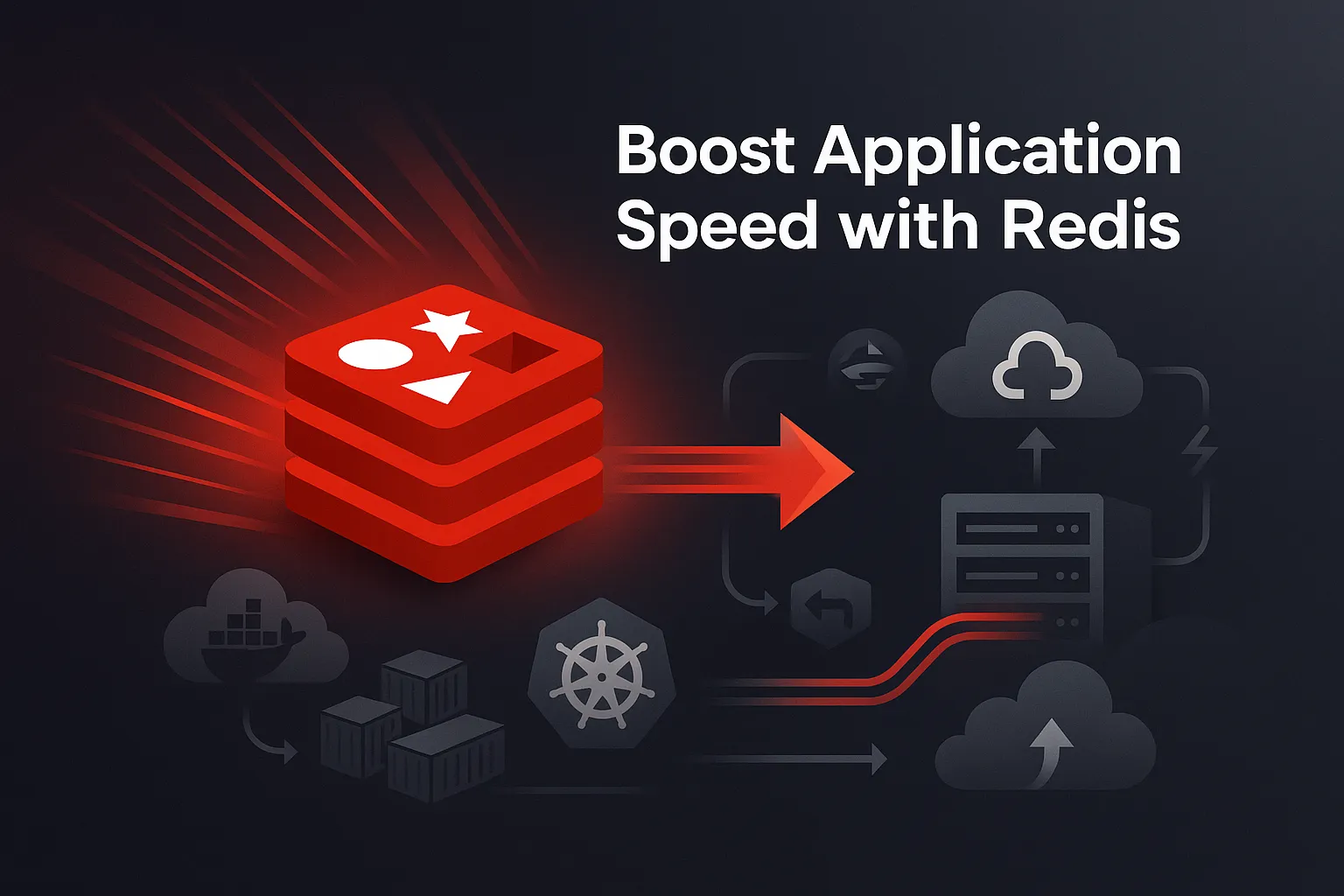
Imagine your users clicking a button and waiting… and waiting… while your application retrieves data from a database. Now imagine the same scenario, but the response comes back almost instantly. That’s the power of caching, and Redis is one of the most effective tools to make this happen. In this guide, we’ll explore how Redis caching can transform your application’s performance from sluggish to lightning-fast, focusing on practical benefits rather than just technical implementation.
The Performance Problem Redis Solves
Before we dive into Redis, let’s understand the core performance challenges in modern applications:
Why Applications Slow Down
- Database bottlenecks: Every database query takes time, especially as your data grows
- Repeated computations: Many applications waste resources recalculating the same results
- Network latency: Round trips to databases or APIs add significant delays
- High traffic spikes: Servers struggle when many users request the same data simultaneously
When your application serves the same data repeatedly (product details, user profiles, search results), fetching it from the source each time creates unnecessary delays. These delays compound as traffic increases, potentially leading to system failures under load.
How Redis Caching Supercharges Performance
Redis works as a high-speed memory cache that sits between your application and your slower data sources. Here’s why it’s so effective at boosting performance:
- Lightning-fast responses: By storing data in memory, Redis delivers responses in microseconds instead of milliseconds (100-1000x faster than disk-based solutions)
- Reduced database load: By serving frequent requests from cache, Redis can reduce database queries by 80-95%
- Improved scalability: Your application can handle more users without proportionally increasing infrastructure
- Consistent speed under load: Performance remains stable even during traffic spikes
Getting Started with Redis Caching
Redis installation is straightforward (using package managers like apt or brew), and connecting it to your application requires minimal code changes. Instead of focusing on technical setup, let’s understand the core strategies that deliver the biggest performance improvements.
Three Simple Caching Strategies That Boost Performance
Let’s explore the most effective ways to implement Redis caching for maximum performance gains:
1. Read-Through Caching: Eliminating Database Bottlenecks
The Performance Problem: Database queries are often the biggest bottleneck in applications, especially for frequently accessed data like product information, user profiles, or configuration settings.
The Caching Solution: With read-through caching, your application first checks Redis for the data. Only when the data isn’t found does it query the database, then stores the result in Redis for future requests.
Performance Impact:
- Reduces database load by up to 95%
- Cuts response times from hundreds of milliseconds to just a few milliseconds
- Database server CPU usage often drops by 50-70%
- Can handle 10x more concurrent users with the same infrastructure
2. Write-Through Caching: Keeping Data Consistent
The Performance Problem: When data is updated, cache and database can become out of sync, causing bugs or requiring cache invalidation that hurts performance.
The Caching Solution: When updating data, write to both the database and the cache simultaneously, ensuring they stay in sync.
Performance Impact:
- Maintains consistently fast read performance even after updates
- Eliminates the “cache miss spike” after content changes
- Prevents serving stale data that could negatively impact user experience
- Simplifies architecture by eliminating complex invalidation logic
3. Predictive Caching: Anticipating User Needs
The Performance Problem: Even with general caching, the first user to request specific content experiences slowness while the cache is being populated.
The Caching Solution: Proactively cache content that users are likely to need based on patterns, trends, or scheduled events.
Performance Impact:
- Delivers consistent performance even for first-time requests
- Handles traffic spikes gracefully (e.g., during sales events or product launches)
- Creates a smoother experience during predictable high-traffic periods
- Allows controlled preloading during off-peak hours to optimize resource usage
Strategic Caching for Maximum Performance Impact
The most effective performance improvements come from strategically identifying what to cache and when. Here’s how to approach this for different parts of your application:
Key Areas to Target for Performance Gains
Applying caching to these high-impact areas typically yields the biggest performance improvements:
- Database Query Results
- Performance Problem: Complex queries joining multiple tables can take seconds to execute
- Performance Solution: Cache query results for 5-15 minutes depending on data volatility
- Impact: Often reduces page load times by 70-90%
- User Session Data
- Performance Problem: Repeated database lookups for the same user data on every request
- Performance Solution: Store session data in Redis with expiration matching session lifetime
- Impact: Reduces authentication overhead and speeds up every authenticated request
- API Responses
- Performance Problem: External APIs are slow, unreliable, or have rate limits
- Performance Solution: Cache API responses with TTLs aligned to data freshness requirements
- Impact: Creates consistent performance even when third-party services slow down
- Computed Results
- Performance Problem: Complex calculations (recommendations, analytics, etc.) repeated unnecessarily
- Performance Solution: Store calculation results in Redis with appropriate TTL
- Impact: Can turn multi-second operations into sub-millisecond responses
Layered Caching Strategy for Optimal Performance
For enterprise applications handling significant traffic, implementing multiple caching layers delivers the best performance:
Layer 1: Browser Cache
- Caches static assets and responses directly in users’ browsers
- Virtually eliminates network time for repeated visits
- Controlled through HTTP cache headers
Layer 2: CDN Cache
- Distributes cached content geographically close to users
- Reduces network latency significantly
- Perfect for static content and semi-dynamic pages
Layer 3: Application Cache (Redis)
- Stores frequently accessed dynamic data
- Eliminates database and computation bottlenecks
- Enables sub-100ms response times for dynamic content
Layer 4: Database Query Cache
- Optimizes repeated identical queries
- Reduces load on database servers
- Complements rather than replaces Redis caching
Advanced Performance Techniques
These sophisticated caching approaches can further enhance performance for high-traffic applications:
- Staggered Expirations
- Instead of having all cache items expire simultaneously (causing traffic spikes), add random time offsets
- This prevents “thundering herd” problems where database servers get overwhelmed
- Results in more consistent performance during cache refreshes
- Fragment Caching
- Cache individual parts of pages separately with different expiration times
- Frequently changing elements (like stock prices) can have short TTLs
- Stable elements (like product descriptions) can have longer TTLs
- Creates maximum cache efficiency while maintaining data freshness
- Background Refresh
- Proactively refresh cache items before they expire
- Users continue receiving fast responses during refresh
- Eliminates the performance penalty for the user who triggers a cache miss
Performance Challenges and Solutions
Even with the best caching strategy, you may encounter performance challenges. Here are common ones with practical solutions:
1. Slow Response Despite Caching
Performance Problem: Some operations remain slow despite implementing Redis.
Performance Solutions:
- Analyze cache hit rates - Low hit rates mean your caching strategy needs refinement
- Review cache key design - Poorly designed keys lead to unnecessary cache misses
- Check cache timeouts - Excessively short TTLs can defeat the purpose of caching
- Monitor Redis server resources - CPU or network bottlenecks can impact Redis itself
2. Performance Spikes and Inconsistency
Performance Problem: Application performs well sometimes but has random slowdowns.
Performance Solutions:
- Implement staggered expirations - Add random time to TTLs to prevent mass expirations
- Use background refresh - Update cache items before they expire
- Monitor database load - Look for correlation between cache misses and database spikes
- Implement circuit breakers - Protect databases from excessive load during cache misses
3. Growing Memory Usage
Performance Problem: Redis memory consumption grows continuously, potentially affecting stability.
Performance Solutions:
- Set appropriate memory limits - Configure Redis to use a fixed maximum amount of memory
- Choose proper eviction policies - Let Redis automatically remove least-used items when memory fills
- Review TTL strategy - Ensure all cache entries have reasonable expiration times
- Monitor key count and size - Identify and optimize unexpectedly large cached items
4. Diminishing Returns from Caching
Performance Problem: Initial gains were impressive, but adding more caching doesn’t yield further improvements.
Performance Solutions:
- Focus on the critical path - Identify the remaining bottlenecks in your request flow
- Look beyond caching - Consider query optimization, code efficiency, or architecture changes
- Implement profiling - Use tools to identify exactly where time is spent in slow requests
- Consider distributed caching - For very large applications, implement Redis Cluster for scale
Measuring the Performance Impact
To quantify the benefits of your Redis implementation and continuously improve:
Essential Performance Metrics to Track
- Response time improvement: Compare before/after response times for key operations
- Server load reduction: Measure database CPU and I/O before and after caching
- Cache effectiveness: Monitor hit rate percentage (aim for >80% for optimal performance)
- Cost savings: Calculate infrastructure cost reduction from improved efficiency
- User experience metrics: Track improvements in bounce rates, conversion rates, etc.
Beyond Caching: Other Redis Performance Boosters
While caching is Redis’s most common performance use case, it offers several other capabilities that can enhance application speed and responsiveness:
- Session Management: Store user sessions for 100x faster access than database storage
- Rate Limiting: Protect your APIs from abuse without impacting performance
- Real-time Analytics: Track metrics instantly without slowing down core operations
- Message Broker: Decouple components for better scalability and fault tolerance
- Task Queue: Process background work efficiently without blocking user interactions
- Leaderboards and Rankings: Calculate and display real-time rankings instantly
- Geospatial Operations: Perform location-based queries with exceptional speed
Each of these capabilities deserves its own article, which we’ll explore in future posts.
Conclusion: The Redis Performance Advantage
Adding Redis caching to your application is one of the highest-impact, lowest-effort performance improvements you can make. The benefits are clear and compelling:
- Dramatically faster response times - Often 5-10x improvement in user-facing speed
- Significantly reduced infrastructure costs - Lower database and server requirements
- Better scalability - Handle more users without proportional cost increases
- Improved reliability - Reduce failure points during traffic spikes
- Enhanced user experience - Deliver the instant responses today’s users expect
The most successful implementations start with high-impact areas (like product pages, user profiles, or search results) and gradually expand caching across the application. By monitoring performance metrics and user experience indicators, you can continuously refine your caching strategy for maximum benefit.
Redis caching isn’t just a technical optimization—it’s a business advantage. Faster applications mean happier users, higher conversion rates, better SEO rankings, and ultimately, more successful digital products.
Have you implemented Redis in your applications? What performance improvements did you see? Share your experience in the comments below.
Have you implemented Redis caching in your applications? What challenges did you face and what benefits did you see? Share your experiences in the comments below.
Comments